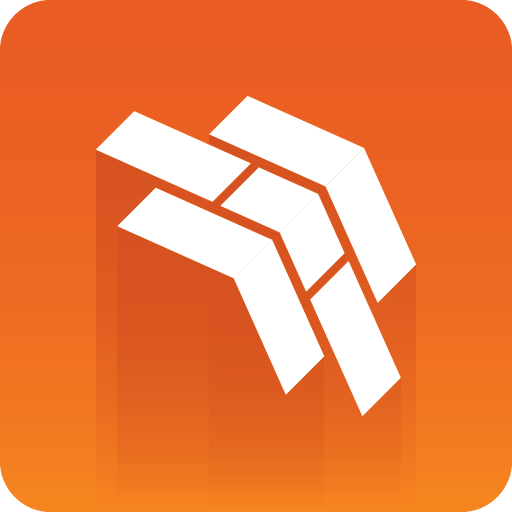
Template Builder User Guide
Version 2.4 | Published November 17, 2022 ©
Form Customization Scripts
If dynamic or advanced customization of the fill-in form is needed, Pilot Edge allows this through template scripting.
Scripts are written in TypeScript, and access the template via a provided Script API named vizrt.
It allows users to customize templates look and behavior, as well as fill in values from external sources. This section describes how to use the script editor, and access the values of the fields supported in Template Builder.
Check out the Quick Start Examples for some quick hands-on experience.
Note: For testing API endpoints, please use the following:
-
HTTP://<PDS-HOST>:8177/testing/fakepremierleague/
-
HTTP://<PDS-HOST>:8177/testing/fakepersonsearch/
These are the following topics:
The Script Editor
The script editor is available in the "Fill-in Form" tab of Template Builder.
It can be used docked or undocked from the Template Builder. While undocked, you can adjust the size of the window for a smoother experience.
There is a search option to search within the script code, and you can access it by clicking the icon
within the script editor.
The script editor also provides error messages depending on the problem. It will show compile or run time errors.
Compile Error
Runtime Error
When trying to catch a runtime error, it is recommend that you name a function with a string value. By naming a function, this name is used in the error message to indicate where the error came from.
In the following example, if you catch an error, the string used under "user defined name" is shown in the error message:
In this case, you can just add the string value in your function error report so it shows in the error message:
Field Access
When using the scripting tool in the template, the individual fields must be accessed through the global name space vizrt.fields (for example, vizrt.fields.$singleline.value).
Note: Writing $singleline.value instead of vizrt.fields.$singleline.value will not work, and will give a Compile Error.
The script executes when a graphic element is opened or created with the scripted template in Pilot Edge.
In Template Builder, the script is also re-loaded and restarted when there are changes made to it.
By typing vizrt.fields, the editor's autocomplete will show you the available fields to choose from.
You can read and write field values, as well as react to value changes from outside the script.
You can also access the properties read-only and hidden of the vizrt fields.
-
onChanged: A property on fields that you can set as a function, and if you do so, this function is called whenever the value of the fields changes, and gets the new value as an argument . If this is not set, it will be null.
Note: Changes done to field values by the template script will not trigger the onChanged function to be called.
-
readOnly: Read and write boolean access, to whether the field should be editable in the form or not. If false , the field and its input elements are editable in the UI. If true , they are read-only and greyed out in the UI, but are accessible, saved and loaded as part of the payload.
-
hidden: Read and write boolean access, to whether the field should be editable in the form or not. If false , the field and its input elements are present and visible in the UI. If true , they are hidden from the UI but are accessible, saved and loaded as part of the payload.
Note: Dashes cannot be used in Typescript with the dot syntax, instead you can use vizrt.fields["$01-week"] syntax to be able to access it.
External Sources
Whether on template load, or as a reaction to a field change, you can initiate HTTP, HTTPS or REST calls to fetch values from third party or external services.
This can easily be done via the browser's built-in fetch API: https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API.
See the Quick Start Examples section for a short example of a REST call triggered by an onChanged event.
Image Metadata
Every image has some amount of metadata attached to it, and with this field you are able to access this metadata by using the script editor.
You can upload images and the corresponding metadata to asset source servers. By accessing an image's metadata, you can auto-fill the name field within a template or alternatively display or hide an image that might be copyrighted.
Note: Image scripting metadata currently works for images retrieved from the following asset source servers: Vizone, Vos, GH and OMS.
How to Use the Image Metadata
If you are using a newly created template, simply choose any image, and then query that image's metadata in the script editor by querying the image's metadata map. With an existing template, which already contains an image that was created before the 2.4 version of Template Builder and Pilot Edge, then simply click on the current image and re-select it from the asset selector, or alternatively select another random image and then select back the original one. Once this is done, you can proceed by querying the image's metadata map.
This example checks if imageName has a metadata key named test and then tries to get the value of that metadata key. You can use the script syntax shown below:
let hasMetadataKey: boolean = vizrt.fields.$imageName.metadata.has(
"test"
)
//true if the metadata contains the key test
let metadataValue: string = vizrt.fields.$imageName.metadata.get(
"test"
)
//it is set to the value it has in the metadata, otherwise it is undefined
The following example shows a more realistic code example of the scripting metadata functionality. An image field named image1 tries to get the metadata value for description and put it into a text field called img1_txt in case it is found, otherwise a message shows explaining it was not found.
if
(vizrt.fields.$image1 != undefined && vizrt.fields.$image1.metadata != undefined) {
let keyName =
"description"
let a = vizrt.fields.$image1.metadata.get(keyName)
// get the metadata value that has the given key
if
(a != undefined) {
vizrt.fields.$img1_txt.value = a
// if the value is not undefined, then set it into a string field within the template
}
else
{
vizrt.fields.$img1_txt.value =
"The key '"
+ keyName +
"' was not found inside the metadata map"
// Alternatively, set it to nothing: vizrt.fields.$img1_txt.value = ""
}
}
This code example will react to any image changes. When the image2 field gets assigned a new image, it tries to get the description from the metadata associated with the new image, into the text field img2_txt. If the description does not exist, a message displays explaining it was not found.
vizrt.fields.$image2.onChanged = () => {
if
(vizrt.fields.$image2.metadata != undefined) {
let keyName =
"description"
let a = vizrt.fields.$image2.metadata.get(keyName)
// get the metadata value that has the given key
if
(a != undefined) {
vizrt.fields.$img2_txt.value = a
// if the value is not undefined, then set it into a string field within the template
}
else
{
vizrt.fields.$img2_txt.value =
"The key '"
+ keyName +
"' was not found inside the metadata map"
// Alternatively, set it to nothing: vizrt.fields.$img2_txt.value = ""
}
}
}
To access the entire unprocessed/unparsed metadata file, use wholeMetadataString. This can be useful for debugging, or for finding the keys available in the metadata:
let rawMetadata:string = vizrt.fields.$imageName.metadata.get(
"wholeMetadataString"
)
Image Metadata XML
An image's metadata is stored within asset source servers in XML format, and
the XML metadata structure should follow a simple field-value (key-value) structure. This is to guarantee that all the metadata is correctly mapped and made accessible through the script editor.
However, since many image metadata XMLs are disorderly, a parser has been created to handle most XML structures, although this has some consequences that should be made aware of. All these example scripts are based on an image field named "image1".
Empty field-value pairs get added accordingly:
<field name=
"car"
/>
<field name=
"color"
>
<value/>
</field>
// Accessing these can be done using:
let a = vizrt.fields.$image1.metadata.get(
"car"
)
let b = vizrt.fields.$image1.metadata.get(
"color"
)
// Both a and b will be ""
Nested structures get stored based on their hierarchy, with "/" being the parent-child separator:
<field name=
"access-rights"
>
<field name=
"user-rights"
>
<value>
true
</value>
</field>
</field>
// To access the user-rights value:
let a = vizrt.fields.$image1.metadata.get(
"access-rights/user-rights"
)
// a will then be set to true.
Any fields with duplicate names get assigned a unique name with an incrementing suffix:
<field name=
"file-link-id"
>
<value>id123</value>
</field>
<field name=
"file-link-id"
>
<value>id456</value>
</field>
<field name=
"file-link-id"
>
<value>id789</value>
</field>
Access duplicate names like this using the incrementing suffix:
let a = vizrt.fields.$image1.metadata.get(
"file-link-id"
)
let b = vizrt.fields.$image1.metadata.get(
"file-link-id(2)"
)
let c = vizrt.fields.$image1.metadata.get(
"file-link-id(3)"
)
a will be
"id123"
b will be
"id456"
c will be
"id789"
Read Only Fields
Some fields are currently supported only for read-access by the scripting API. These are the following:
-
Duplet
-
Triplet
-
Map
-
Image
-
Video
For the Image and Video fields, the script is able to access some properties (their height, width, etc.) from the file.
The following example shows how to retrieve the image height:
vizrt.fields.$ImageInfo.value =
"No image info"
;
vizrt.fields.$image.onChanged =() => {
vizrt.fields.$ImageInfo.value =
'Image changed'
;
var v = vizrt.fields.$image.value;
if
(v != undefined && v.height != undefined)
vizrt.fields.$ImageInfo.value = v.height.toString();
else
vizrt.fields.$ImageInfo.value =
"No image info"
;
}
Note: Because images and videos can be undefined, they must be checked before they are used.
Unsupported Fields
As of now, all List and Table fields are unavailable from the scripting API.